Previously, we learned how to create an object (a line with fixed length) via the PythonParts API. Today I’m going to show you how to set up handles for our HelloWorld script…
1) GUI Script
The handle that we are going to create will allow us to interact with a variable called Length.
So we need to create it in the palette.
I take my HelloWorld.pyp file and, between the Element tags, I first generate a page :
<Page>
<Name>Page1</Name>
<Text>Général</Text></Page>
Please note :
- the pages corresponding to the different tabs in the palette ;
- we give it a name Page1 and I place some text that shows up in Allplan : Général ;
- the pages’ name is displayed from the 2nd tab.
Then, into my Page1, I place the fields to insert my variable :
<Parameter>
<Name>LineLength</Name>
<Text>Longueur</Text>
<Value>1000.0</Value>
<ValueType>Length</ValueType>
</Parameter>
Let’s look at it :
-
Parameter
Required tag, it’s the source of all our variables.
-
Name
The ID, it must be unique because it will be called in our main scripts.
-
Text
The text that is displayed in Allplan.
-
Value
We set a default value for our variable, here “1000”.
-
ValueType
Finally, the type of variable we want to use.
Please note :
- there is a lot of possibilities (integer, list, …) ; feel free to look at the PythonPart AllControls (C:\ProgramData\Nemetschek\Allplan\2023\Etc\Examples\PythonParts\PaletteExamples) or this link ;
- Name (must be unique), Text and ValueType are the 3 required fields for any parameter.
Here is the complete source code :
2) Main Script
We start by import the needed modules into our Python script :
from HandlePropertiesService import HandlePropertiesService
from HandleDirection import HandleDirection
from HandleParameterData import HandleParameterData
from HandleParameterType import HandleParameterType
from HandleProperties import HandleProperties
We create a new function move_handle that will listen to any modification on the handle :
def move_handle(build_ele, handle_prop, input_pnt, doc):
HandlePropertiesService.update_property_value(build_ele, handle_prop, input_pnt)
return create_element(build_ele, doc)
I go into my create_element function, and I initialize my list of handles :
handle_list = []
Don’t forget to add this list to my return statement :
return CreateElementResult(model_ele_list, handle_list)
Then, I extract the informations from the palette :
# Extract parameters values from palette
line_length = build_ele.LineLength.value
Please note : here is the Name field of the GUI script.
I modify my 2D line by replacing the fixed length (1000) by my variable line_length :
# Create 2D line
line = Geometry.Line2D(0, 0, line_length, 0)
Finally, I create my handle :
# Create the handles
handle_length = HandleProperties(« LineLengthHandle »,
Geometry.Point3D(line_length, 0, 0),
Geometry.Point3D(),
[HandleParameterData(« LineLength », HandleParameterType.POINT_DISTANCE)],
HandleDirection.X_DIR
)
handle_length.info_text = « Longueur »
handle_list.append(handle_length)
Let’s look in detail :
- first I name this variable : handle_length ;
- I give an ID for my handle LineLengthHandle ;
- I set the final point Geometry.Point3D(line_length, 0, 0) ;
- I set the first point (Geometry.Point3D() ;
- I associate it with my LineLength variable of the GUI script ;
- I freeze the direction along the X axis with HandleDirection.X_DIR ;
- for a better user experience I can add a text to my handle (displayed in Allplan) ;
- Finally, I add my handle to my set with handle_list.append(handle_length).
Here is the complete source code :
My simple line became interactive with an input field for the length and my handle is now functional :
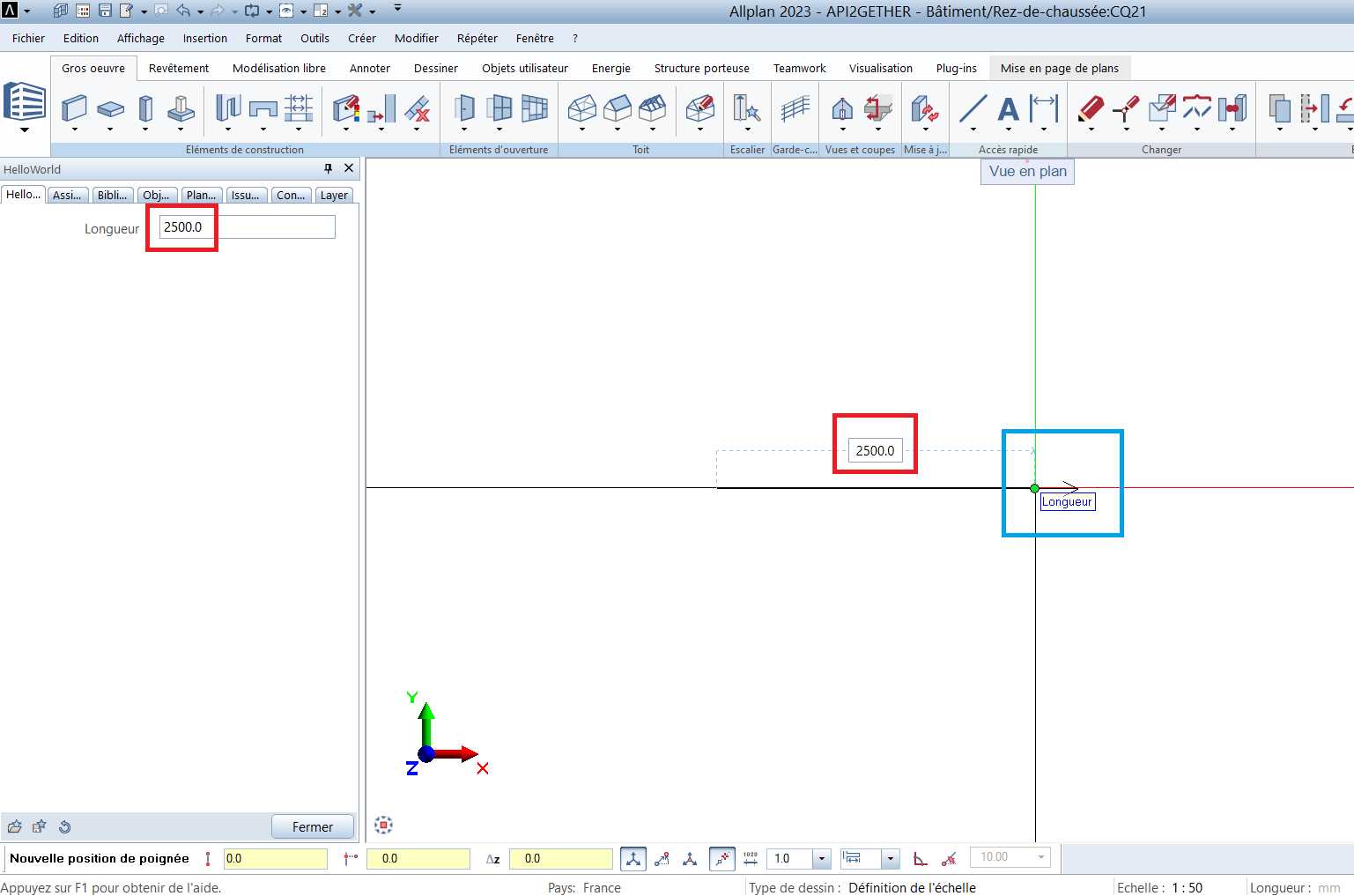
0 Comments